I’m trying to accomplish a Horizontal Scrollable Menu by using the following Markup:
<Panel Dock="Top" Height="50" Background="{data.market.color}">
<ScrollView AllowedScrollDirections="Horizontal">
<Grid ColumnCount="14" RowCount="1" Padding="10, 0, 10, 0">
<Panel Width="150" Margin="0, 0, 0, 0" Alignment="Center">
<Text Font="MaterialIcons" Color="White" Alignment="Center" FontSize="22"></Text>
<Text Font="Roboto" Color="White" Alignment="Center" FontSize="16" Margin="70, 0, 0, 0">Menú</Text>
</Panel>
<Panel Width="150" Margin="70, 0, 0, 0" Alignment="Center">
<Text Font="MaterialIcons" Color="White" Alignment="Center" FontSize="22"></Text>
<Text Font="Roboto" Color="White" Alignment="Center" FontSize="16" Margin="70, 0, 0, 0">Menú</Text>
</Panel>
<Panel Width="150" Margin="110, 0, 0, 0" Alignment="Center">
<Text Font="MaterialIcons" Color="White" Alignment="Center" FontSize="22"></Text>
<Text Font="Roboto" Color="White" Alignment="Center" FontSize="16" Margin="70, 0, 0, 0">Menú</Text>
</Panel>
</Grid>
</ScrollView>
</Panel>
However, the output is the following:

As you can see, I have to specify to each Panel a left margin in order for me to be able to adjust them correctly in my grid. Is there an easier way to do this? I don’t need 14 Columns on my Grid, but I would daresay I need at least 6 and I put it inside a ScrollView so I am able to scroll it sideways.
Hey @yisera.
You could use StackPanel
instead of Grid
. You can stack items horizontally and scroll through items in ScrollView
.
A modified code should look something like this:
<Panel Dock="Top" Height="50" Background="{data.market.color}">
<ScrollView AllowedScrollDirections="Horizontal">
<StackPanel Padding="10, 0, 10, 0" Orientation="Horizontal">
<Panel Width="150" Alignment="Center">
<Text Font="MaterialIcons" Color="White" Alignment="Center" FontSize="22"></Text>
<Text Font="Roboto" Color="White" Alignment="Center" FontSize="16" Margin="70, 0, 0, 0">Menú</Text>
</Panel>
<Panel Width="150" Alignment="Center">
<Text Font="MaterialIcons" Color="White" Alignment="Center" FontSize="22"></Text>
<Text Font="Roboto" Color="White" Alignment="Center" FontSize="16" Margin="70, 0, 0, 0">Menú</Text>
</Panel>
<Panel Width="150" Alignment="Center">
<Text Font="MaterialIcons" Color="White" Alignment="Center" FontSize="22"></Text>
<Text Font="Roboto" Color="White" Alignment="Center" FontSize="16" Margin="70, 0, 0, 0">Menú</Text>
</Panel>
</StackPanel>
</ScrollView>
</Panel>
Hope this helps.
With a little bit more of readjustment, it works fine now!
One more request @Arturs if you know, how can I make the Panel hide sliding up when the user scrolls down?
Here’s what I want to accomplish:
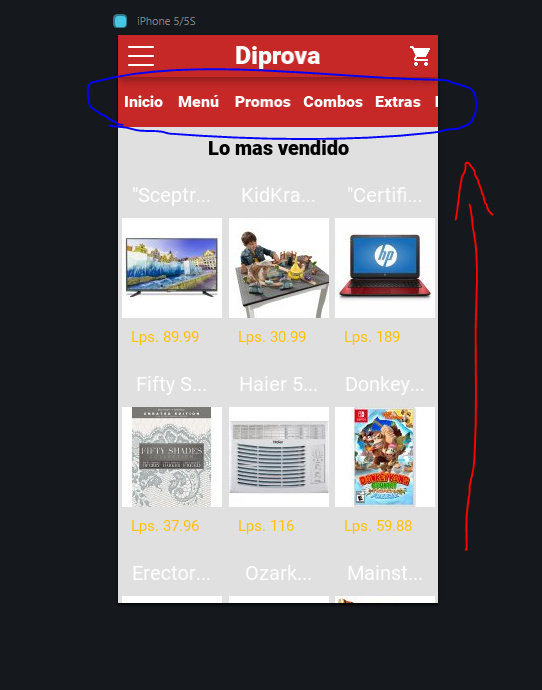
When the user scrolls to see more products, I want to be able to make the Panel slide up and hide until the user scrolls on the opposite direction.
Do you know a way to manage this?
Hey @yisera.
I am glad it worked out for you. 
To hide element in ScrollView
you can use ScrollingAnimation.
Yup, kinda works with the following code:
<ScrollingAnimation From="0" To="1">
<Move Target="ClientSubmenu" Y="-1.2" RelativeTo="Size" RelativeNode="MainNavBar" />
</ScrollingAnimation>
However like the docs say, Move
doesn’t affect layout so it does hide but the space doesn’t shrink which is also a desired behavior I would like to achieve.
Also, when Scrolling down, it disappears but when scrolling up it waits until it reaches to top to display the bar again which isn’t the point. Any idea how can I fix both of these issues?
Hey @yisera.
You can use LayoutAnimation to change width or height of an element in the layout.
To animate elements, while user scrolls you can use WhileScrollable, WhileScrolled or WhileInteracting.