Thanks,
this is all very confusing, to say the least =(
What I discovered now, if I make the change as you said above:
I have a TextView to display the jsProgress:
<TextView ux:Name="tv1" Value="{jsProgress}" TextColor="#fff" Width="50" Height="20" Alignment="TopLeft"/>
then I have the timeline:
<Timeline ux:Name="timeline" Progress="{jsProgress}" >
and then the js code:
<JavaScript>
var Observable = require("FuseJS/Observable");
var jsProgress = Observable(0);
module.exports = {
jsProgress : jsProgress
};
</JavaScript>
And lastly, the button to start the animation:
<MyButton ux:Name="btnStart" Text="START" Width="100" Height="100">
<Clicked>
<Set timeline.TargetProgress="1" />
</Clicked>
</MyButton>
What happens if I press the button is that the animation “steps” through the animation, it doesnt smoothly go from start to finish. first time I click, it looks like this:
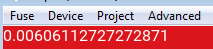
So, its on Progress 0.006
If I keep pressing, eventually the animated stuff comes into view:
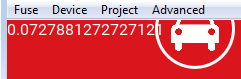
This behaviour disappears if I remove the textview_
<TextView ux:Name="tv1" Value="{jsProgress}" TextColor="#fff" Width="50" Height="20" Alignment="TopLeft"/>
Is this expected behaviour? Why? Isnt the textview just displaying the value?